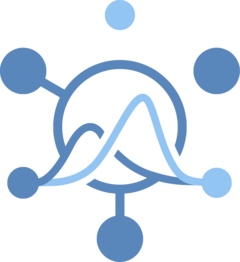
Basic Plot for model outputs
Source:R/plot_step_ahead_model_output.R
plot_step_ahead_model_output.Rd
Create a simple Plotly time-series plot for model projection outputs.
Usage
plot_step_ahead_model_output(
model_out_tbl,
target_data,
use_median_as_point = FALSE,
show_plot = TRUE,
plot_target = TRUE,
x_col_name = "target_date",
x_target_col_name = "date",
show_legend = TRUE,
facet = NULL,
facet_scales = "fixed",
facet_nrow = NULL,
facet_ncol = NULL,
facet_title = "top left",
interactive = TRUE,
fill_by = "model_id",
pal_color = "Set2",
one_color = "blue",
fill_transparency = 0.25,
intervals = c(0.5, 0.8, 0.95),
top_layer = "model_output",
title = NULL,
ens_color = NULL,
ens_name = NULL,
group = NULL
)
Arguments
- model_out_tbl
a
model_out_tbl
object, containing all the required columns including a column containing date information (x_col_name
parameter) and a columnvalue
.- target_data
a
data.frame
object containing the target data, with a column containing date information (x_target_col_name
parameter) and a columnobservation
. Ignored, ifplot_target = FALSE
.- use_median_as_point
a
Boolean
for using median quantile as point in plot. Default to FALSE. If TRUE, will select first anymedian
output type value and if nomedian
value included inmodel_out_tbl
; will selectquantile = 0.5
output type value.- show_plot
a
boolean
for showing the plot. Default to TRUE.- plot_target
a
boolean
for showing the target data in the plot. Default to TRUE. Data used in the plot comes from the parametertarget_data
- x_col_name
column name containing the date information for
all_plot
andall_ens
data frames, value will be map to the x-axis of the plot. By default, "target_date".- x_target_col_name
column name containing the date information for
target_data
data frame, value will be map to the x-axis of the plot. By default, "date".- show_legend
a
boolean
for showing the legend in the plot. Default to TRUE.- facet
a unique value corresponding as a task_id variable name (interpretable as facet option for ggplot)
- facet_scales
argument for scales as in ggplot2::facet_wrap or equivalent to
shareX
,shareY
in plotly::subplot. Default to "fixed" (x and y axes are shared).- facet_nrow
a numeric, number of rows in the layout.
- facet_ncol
a numeric, number of columns in the layout (ignored in plotly::subplot)
- facet_title
a
string
, position of each subplot tile (value associated with thefacet
parameter). "top right", "top left" (default), "bottom right", "bottom left" are the possible values,NULL
to remove the title. For interactive plot only.- interactive
a
boolean
to output an "interactive" version of the plot (using Plotly) or a "static" plot (using ggplot2). By default,TRUE
(interactive plot)- fill_by
name of a column for specifying colors and legend in plot. The
pal_color
parameter can be use to change the palette. Default tomodel_id
.- pal_color
a
character
string for specifying the palette color in the plot. Please refer toRColorBrewer::display.brewer.all()
. IfNULL
, onlyone_color
parameter will be used for all models. Default to"Set2"
- one_color
a
character
string for specifying the color in the plot ifpal_color
is set toNULL
. Please refer tocolors()
for accepted color names. Default to"blue"
- fill_transparency
numeric value used to set transparency of intervals. 0 means fully transparent, 1 means opaque. Default to
0.25
- intervals
a vector of
numeric
values indicating which central prediction interval levels to plot.NULL
means no interval levels. If not provided, it will default toc(.5, .8, .95)
. When plotting 6 models or more, the plot will be reduced to show.95
interval only. Value possibles:0.5, 0.8, 0.9, 0.95
- top_layer
character vector, where the first element indicates the top layer of the resulting plot. Possible options are
"model_output"
(default) and"target"
- title
a
character
string, if not NULL, will be added as title to the plot- ens_color
a
character
string of a color name, if not NULL, will be use as color for the model name associated with the parameterens_name
(both parameter need to be provided)- ens_name
a
character
string of a model name, if not NULL, will be use to change the color for the model name, associated with the parameterens_color
(both parameter need to be provided)- group
column name for partitioning the data in the data according the the value in the column. Please refer to ggplot2::aes_group_order for more information. By default, NULL (no partitioning).
Examples
# Load and Prepare Data
# The package hubExmaple contains example files, please consult the
# documentation associated with the package, for more information.
library(hubExamples)
head(scenario_outputs)
#> # A tibble: 6 × 9
#> model_id origin_date scenario_id location target horizon output_type
#> <chr> <date> <chr> <chr> <chr> <int> <chr>
#> 1 HUBuni-simexamp 2021-03-07 A-2021-03-05 US inc case 1 quantile
#> 2 HUBuni-simexamp 2021-03-07 A-2021-03-05 US inc case 1 quantile
#> 3 HUBuni-simexamp 2021-03-07 A-2021-03-05 US inc case 1 quantile
#> 4 HUBuni-simexamp 2021-03-07 A-2021-03-05 US inc case 1 quantile
#> 5 HUBuni-simexamp 2021-03-07 A-2021-03-05 US inc case 1 quantile
#> 6 HUBuni-simexamp 2021-03-07 A-2021-03-05 US inc case 1 quantile
#> # ℹ 2 more variables: output_type_id <dbl>, value <dbl>
head(scenario_target_ts)
#> # A tibble: 6 × 4
#> location date observation target
#> <chr> <chr> <int> <chr>
#> 1 US 2020-10-03 300678 inc case
#> 2 US 2020-10-10 334493 inc case
#> 3 US 2020-10-17 388282 inc case
#> 4 US 2020-10-24 484422 inc case
#> 5 US 2020-10-31 571389 inc case
#> 6 US 2020-11-07 776479 inc case
projection_data <- dplyr::mutate(scenario_outputs,
target_date = as.Date(origin_date) + (horizon * 7) - 1)
projection_data <- dplyr::filter(projection_data,
scenario_id == "A-2021-03-05", location == "US")
projection_data <- hubUtils::as_model_out_tbl(projection_data)
target_data_us <- dplyr::filter(scenario_target_ts, location == "US",
date < min(projection_data$target_date) + 21,
date > "2020-10-01")
# Plot
plot_step_ahead_model_output(projection_data, target_data_us)