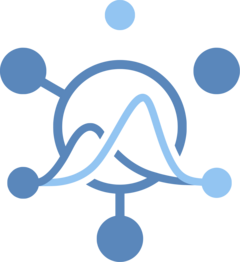
Validate a hub config file against a Infectious Disease Modeling Hubs schema
Source:R/validate_config.R
validate_config.Rd
Validate a hub config file against a Infectious Disease Modeling Hubs schema
Usage
validate_config(
hub_path = ".",
config = c("tasks", "admin"),
config_path = NULL,
schema_version = "from_config",
branch = "main"
)
Arguments
- hub_path
Path to a local hub directory.
- config
Name of config file to validate. One of
"tasks"
or"admin"
.- config_path
Defaults to
NULL
which assumes all config files are in thehub-config
directory in the root of hub directory. Argumentconfig_path
can be used to override default by providing a path to the config file to be validated.- schema_version
Character string specifying the json schema version to be used for validation. The default value
"from_config"
will use the version specified in theschema_version
property of the config file."latest"
will use the latest version available in the Infectious Disease Modeling Hubs schemas repository. Alternatively, a specific version of a schema (e.g."v0.0.1"
) can be specified.- branch
The branch of the Infectious Disease Modeling Hubs schemas repository from which to fetch schema. Defaults to
"main"
.
Value
Returns the result of validation. If validation is successful, will
return TRUE
. If any validation errors are detected, returns FALSE
with
details of errors appended as a data.frame to an errors
attribute.
To access
the errors table use attr(x, "errors")
where x
is the output of the function.
You can print a more concise and easier to view version of an errors table with
view_config_val_errors()
.
See also
Other functions supporting config file validation:
get_schema()
,
get_schema_url()
,
get_schema_valid_versions()
,
validate_hub_config()
,
view_config_val_errors()
Examples
# Valid config file
validate_config(
hub_path = system.file(
"testhubs/simple/",
package = "hubUtils"
),
config = "tasks"
)
#> Loading required namespace: jsonvalidate
#> ✔ Successfully validated config file /home/runner/work/_temp/Library/hubUtils/testhubs/simple/hub-config/tasks.json against schema <https://raw.githubusercontent.com/Infectious-Disease-Modeling-Hubs/schemas/main/v2.0.0/tasks-schema.json>
#> [1] TRUE
#> attr(,"config_path")
#> /home/runner/work/_temp/Library/hubUtils/testhubs/simple/hub-config/tasks.json
#> attr(,"schema_version")
#> [1] "v2.0.0"
#> attr(,"schema_url")
#> https://raw.githubusercontent.com/Infectious-Disease-Modeling-Hubs/schemas/main/v2.0.0/tasks-schema.json
# Config file with errors
config_path <- system.file("error-schema/tasks-errors.json",
package = "hubUtils"
)
validate_config(config_path = config_path, config = "tasks")
#> Warning: Hub configured using schema version v0.0.0.9. Support for schema earlier than
#> v2.0.0 was deprecated in hubUtils 0.0.0.9010.
#> ℹ Please upgrade Hub config files to conform to, at minimum, version v2.0.0 as
#> soon as possible.
#> Warning: Schema errors detected in config file
#> /home/runner/work/_temp/Library/hubUtils/error-schema/tasks-errors.json
#> validated against schema
#> <https://raw.githubusercontent.com/Infectious-Disease-Modeling-Hubs/schemas/main/v0.0.0.9/tasks-schema.json>
#> [1] FALSE
#> attr(,"errors")
#> instancePath
#> 1 /rounds/0/model_tasks/0/task_ids/target/required
#> 2 /rounds/0/model_tasks/0/output_type/mean
#> 3 /rounds/0/model_tasks/0/output_type/quantile
#> 4 /rounds/0/submissions_due
#> 5 /rounds/0/submissions_due/start
#> 6 /rounds/0/submissions_due/end
#> 7 /rounds/0/submissions_due
#> schemaPath
#> 1 #/properties/rounds/items/properties/model_tasks/items/properties/task_ids/properties/target/properties/required/type
#> 2 #/properties/rounds/items/properties/model_tasks/items/properties/output_type/properties/mean/required
#> 3 #/properties/rounds/items/properties/model_tasks/items/properties/output_type/properties/quantile/required
#> 4 #/properties/rounds/items/properties/submissions_due/oneOf/0/required
#> 5 #/properties/rounds/items/properties/submissions_due/oneOf/1/properties/start/type
#> 6 #/properties/rounds/items/properties/submissions_due/oneOf/1/properties/end/type
#> 7 #/properties/rounds/items/properties/submissions_due/oneOf
#> keyword params.type params.missingProperty params.passingSchemas
#> 1 type array, null <NA> NA
#> 2 required NULL type_id NA
#> 3 required NULL type_id NA
#> 4 required NULL relative_to NA
#> 5 type string <NA> NA
#> 6 type string <NA> NA
#> 7 oneOf NULL <NA> NA
#> message
#> 1 must be array,null
#> 2 must have required property 'type_id'
#> 3 must have required property 'type_id'
#> 4 must have required property 'relative_to'
#> 5 must be string
#> 6 must be string
#> 7 must match exactly one schema in oneOf
#> schema
#> 1 array, null
#> 2 type_id, value
#> 3 type_id, value
#> 4 relative_to, start, end
#> 5 string
#> 6 string
#> 7 Name of task id variable in relation to which submission start and end dates are calculated., NA, string, NA, Difference in days between start and origin date., Submission start date., integer, string, NA, date, Difference in days between end and origin date., Submission end date., integer, string, NA, date, relative_to, start, end, start, end
#> parentSchema.description
#> 1 Array of target unique identifiers that must be present for submission to be valid. Can be null if no targets are required and all valid targets are specified in the optional property.
#> 2 Object defining the mean of the predictive distribution output type.
#> 3 Object defining the quantiles of the predictive distribution output type.
#> 4 <NA>
#> 5 Submission start date.
#> 6 Submission end date.
#> 7 Object defining the dates by which model forecasts must be submitted to the hub.
#> parentSchema.type parentSchema.type
#> 1 array, null string
#> 2 object <NA>
#> 3 object <NA>
#> 4 NULL <NA>
#> 5 string <NA>
#> 6 string <NA>
#> 7 object <NA>
#> parentSchema.properties.type_id.description
#> 1 <NA>
#> 2 type_id is not meaningful for a mean output_type. The property is primarily used to determine whether mean is a required or optional output type through properties required and optional. If mean is a required output type, the required property must be an array containing the single string element 'NA' and the optional property must be set to null. If mean is an optional output type, the optional property must be an array containing the single string element 'NA' and the required property must be set to null
#> 3 Object containing required and optional arrays defining the probability levels at which quantiles of the predictive distribution will be recorded.
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.type_id.examples
#> 1 NULL
#> 2 NA, NA
#> 3 0.25, 0.50, 0.75, 0.10, 0.20, 0.30, 0.40, 0.60, 0.70, 0.80, 0.90
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.type_id.type
#> 1 <NA>
#> 2 object
#> 3 object
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.type_id.oneOf
#> 1 NULL
#> 2 When mean is required, property set to single element 'NA' array, When mean is optional, property set to null, array, null, NA, NA, 1, NA, When mean is required, property set to null, When mean is optional, property set to single element 'NA' array, null, array, NA, NA, NA, 1
#> 3 NULL
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.type_id.required
#> 1 NULL
#> 2 required, optional
#> 3 required, optional
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.type_id.properties.required.description
#> 1 <NA>
#> 2 <NA>
#> 3 Array of unique probability levels between 0 and 1 that must be present for submission to be valid. Can be null if no probability levels are required and all valid probability levels are specified in the optional property.
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.type_id.properties.required.type
#> 1 NULL
#> 2 NULL
#> 3 array, null
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.type_id.properties.required.items.type
#> 1 <NA>
#> 2 <NA>
#> 3 number
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.type_id.properties.required.items.minimum
#> 1 NA
#> 2 NA
#> 3 0
#> 4 NA
#> 5 NA
#> 6 NA
#> 7 NA
#> parentSchema.properties.type_id.properties.required.items.maximum
#> 1 NA
#> 2 NA
#> 3 1
#> 4 NA
#> 5 NA
#> 6 NA
#> 7 NA
#> parentSchema.properties.type_id.properties.optional.description
#> 1 <NA>
#> 2 <NA>
#> 3 Array of valid but not required unique probability levels. Can be null if all probability levels are required and are specified in the required property.
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.type_id.properties.optional.type
#> 1 NULL
#> 2 NULL
#> 3 array, null
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.type_id.properties.optional.items.type
#> 1 <NA>
#> 2 <NA>
#> 3 number
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.type_id.properties.optional.items.minimum
#> 1 NA
#> 2 NA
#> 3 0
#> 4 NA
#> 5 NA
#> 6 NA
#> 7 NA
#> parentSchema.properties.type_id.properties.optional.items.maximum
#> 1 NA
#> 2 NA
#> 3 1
#> 4 NA
#> 5 NA
#> 6 NA
#> 7 NA
#> parentSchema.properties.value.type
#> 1 <NA>
#> 2 object
#> 3 object
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.value.description
#> 1 <NA>
#> 2 Object defining the characteristics of valid mean values.
#> 3 Object defining the characteristics of valid quantiles of the predictive distribution at a given probability level.
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.value.examples
#> 1 NULL
#> 2 numeric, 0
#> 3 NULL
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.value.properties.type.description
#> 1 <NA>
#> 2 Data type of mean values.
#> 3 Data type of quantile values.
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.value.properties.type.type
#> 1 <NA>
#> 2 string
#> 3 string
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.value.properties.type.enum
#> 1 NULL
#> 2 numeric, double, integer
#> 3 numeric, double, integer
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.value.properties.type.examples
#> 1 NULL
#> 2 NULL
#> 3 numeric
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.value.properties.minimum.description
#> 1 <NA>
#> 2 The minimum inclusive valid mean value
#> 3 The minimum inclusive valid quantile value (optional).
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.value.properties.minimum.type
#> 1 <NA>
#> 2 integer
#> 3 number
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.value.properties.minimum.examples
#> 1 NULL
#> 2 NULL
#> 3 0
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.value.properties.maximum.description
#> 1 <NA>
#> 2 the maximum inclusive valid mean value
#> 3 The maximum inclusive valid quantile value (optional).
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.value.properties.maximum.type
#> 1 <NA>
#> 2 integer
#> 3 number
#> 4 <NA>
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.value.required
#> 1 NULL
#> 2 type
#> 3 type
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 NULL
#> parentSchema.properties.relative_to.description
#> 1 <NA>
#> 2 <NA>
#> 3 <NA>
#> 4 Name of task id variable in relation to which submission start and end dates are calculated.
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.relative_to.type
#> 1 <NA>
#> 2 <NA>
#> 3 <NA>
#> 4 string
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.start.description
#> 1 <NA>
#> 2 <NA>
#> 3 <NA>
#> 4 Difference in days between start and origin date.
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.start.type
#> 1 <NA>
#> 2 <NA>
#> 3 <NA>
#> 4 integer
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.end.description
#> 1 <NA>
#> 2 <NA>
#> 3 <NA>
#> 4 Difference in days between end and origin date.
#> 5 <NA>
#> 6 <NA>
#> 7 <NA>
#> parentSchema.properties.end.type parentSchema.required parentSchema.format
#> 1 <NA> NULL <NA>
#> 2 <NA> type_id, value <NA>
#> 3 <NA> type_id, value <NA>
#> 4 integer relative_to, start, end <NA>
#> 5 <NA> NULL date
#> 6 <NA> NULL date
#> 7 <NA> start, end <NA>
#> parentSchema.examples
#> 1 NULL
#> 2 NULL
#> 3 NULL
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 2022-06-07, -4, 2022-07-20, 2, NA, origin_date
#> parentSchema.oneOf
#> 1 NULL
#> 2 NULL
#> 3 NULL
#> 4 NULL
#> 5 NULL
#> 6 NULL
#> 7 Name of task id variable in relation to which submission start and end dates are calculated., NA, string, NA, Difference in days between start and origin date., Submission start date., integer, string, NA, date, Difference in days between end and origin date., Submission end date., integer, string, NA, date, relative_to, start, end, start, end
#> data
#> 1 wk inc flu hosp
#> 2 NA, NA, integer, 0
#> 3 0.01, 0.025, 0.05, 0.1, 0.15, 0.2, 0.25, 0.3, 0.35, 0.4, 0.45, 0.5, 0.55, 0.6, 0.65, 0.7, 0.75, 0.8, 0.85, 0.9, 0.95, 0.975, 0.99, integer, 0
#> 4 -6, 1
#> 5 -6
#> 6 1
#> 7 -6, 1
#> dataPath
#> 1 /rounds/0/model_tasks/0/task_ids/target/required
#> 2 /rounds/0/model_tasks/0/output_type/mean
#> 3 /rounds/0/model_tasks/0/output_type/quantile
#> 4 /rounds/0/submissions_due
#> 5 /rounds/0/submissions_due/start
#> 6 /rounds/0/submissions_due/end
#> 7 /rounds/0/submissions_due
#> attr(,"config_path")
#> [1] "/home/runner/work/_temp/Library/hubUtils/error-schema/tasks-errors.json"
#> attr(,"schema_version")
#> [1] "v0.0.0.9"
#> attr(,"schema_url")
#> https://raw.githubusercontent.com/Infectious-Disease-Modeling-Hubs/schemas/main/v0.0.0.9/tasks-schema.json